Force OneDrive to Synchronize
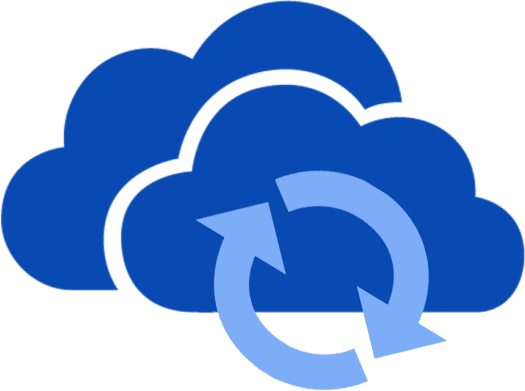
OneDrive is a great way to create backups and keep your files in the cloud and access them wherever you want.
But if you are like me, you don't want to place all your files and documents in the OneDrive folder located in C:\Users\%UserProfile%\OneDrive
because this can take up a lot of disk space, or you like your own folder structures on other drives.
Luckily you can create shortcuts in the OneDrive folder to your actual folder locations by executing the following command in the Command Prompt:
mklink /j "%UserProfile%\OneDrive\My Folder" "D:\Large Files\My Folder"
The downside of that that when a file is added/updated/deleted, it will not trigger a sync from OneDrive as it would when the file was placed directly in the OneDrive folder. The sync is done when OneDrive is started after boot-up, or sometimes at random it would seem.
You can always manually close and open the OneDrive app, but this is not user friendly since you cannot close it the normal way. So I created a simple Console App that writes a file to the OneDrive folder, and then deletes it immediately. The delete will trigger the sync.
Then simply create a Task in Task Scheduler that runs every x minutes to sync your files. Or place the Console App on your desktop to trigger it manually.
c:\files\VDWWD_SyncForcer.exe "C:\Users\USERNAME\OneDrive"
When triggering the App you need to specify the path to your OneDrive folder as a parameter with double quotes.
View source on GitHub
Code Snippets
using System; using System.IO; namespace VDWWD_SyncForcer { class Program { static void Main(string[] args) { //check if there are arguments specified if (args.Length == 0) { Console.WriteLine("Please specify the OneDrive folder."); return; } //get the folder from the arguments collection string folder = args[0].TrimEnd('\\'); //or hardcode a path to your onedrive folder //string folder = @"c:\path_to_onedrive"; //check if the folder exists if (!Directory.Exists(folder)) { Console.WriteLine($"The OneDrive folder \"{folder}\" was not found."); return; } //add a filename string file = folder + @"\ForceSync.txt"; try { //create a text file using (var writer = new StreamWriter(file, true)) { writer.WriteLine("VDWWD"); } //then delete it again File.Delete(file); Console.WriteLine("OneDrive Sync triggered."); } catch (Exception ex) { Console.WriteLine("Error: " + ex.Message); } } } }